Overview
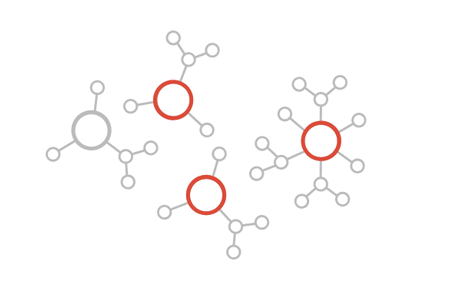
The Freebase Search API provides access to Freebase data given a free text query. The results of the query are ordered and have a numerical relevancy score.
Developers can apply filters to constrain the search results to certain types of data. See the Search Cookbook for more information on how to construct detailed search queries.
Some examples of how developers may want to use the Search API include:
- Autosuggesting entities (e.g. Freebase Suggest Widget)
- Getting a ranked list of the most notable entities with a given name.
- Finding entities using Search Metaschema.
The following code samples in several supported languages show how to perform a search for a musical artist that matches the text “Cee Lo Green”. An additional constraint is that they created something called “The Lady Killer”.
Python
import json import urllib api_key = open(".api_key").read() query = 'blue bottle' service_url = 'https://www.googleapis.com/freebase/v1/search' params = { 'query': query, 'key': api_key } url = service_url + '?' + urllib.urlencode(params) response = json.loads(urllib.urlopen(url).read()) for result in response['result']: print result['name'] + ' (' + str(result['score']) + ')'
Ruby
require 'rubygems' require 'cgi' require 'httparty' require 'json' require 'addressable/uri' API_KEY = open(".freebase_api_key").read() url = Addressable::URI.parse('https://www.googleapis.com/freebase/v1/search') url.query_values = { 'query' => 'Blue Bottle', 'key'=> API_KEY } response = HTTParty.get(url, :format => :json) response['result'].each { |topic| puts topic['name'] }This example uses the Httparty and Addressable libraries.
Java
package com.freebase.samples; import com.google.api.client.http.GenericUrl; import com.google.api.client.http.HttpRequest; import com.google.api.client.http.HttpRequestFactory; import com.google.api.client.http.HttpResponse; import com.google.api.client.http.HttpTransport; import com.google.api.client.http.javanet.NetHttpTransport; import com.jayway.jsonpath.JsonPath; import java.io.FileInputStream; import java.util.Properties; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.JSONParser; public class SearchExample { public static Properties properties = new Properties(); public static void main(String[] args) { try { properties.load(new FileInputStream("freebase.properties")); HttpTransport httpTransport = new NetHttpTransport(); HttpRequestFactory requestFactory = httpTransport.createRequestFactory(); JSONParser parser = new JSONParser(); GenericUrl url = new GenericUrl("https://www.googleapis.com/freebase/v1/search"); url.put("query", "Cee Lo Green"); url.put("filter", "(all type:/music/artist created:\"The Lady Killer\")"); url.put("limit", "10"); url.put("indent", "true"); url.put("key", properties.get("API_KEY")); HttpRequest request = requestFactory.buildGetRequest(url); HttpResponse httpResponse = request.execute(); JSONObject response = (JSONObject)parser.parse(httpResponse.parseAsString()); JSONArray results = (JSONArray)response.get("result"); for (Object result : results) { System.out.println(JsonPath.read(result,"$.name").toString()); } } catch (Exception ex) { ex.printStackTrace(); } } }
Javascript
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script> </head> <body><aside class="warning"><strong>Warning: </strong>The Freebase API will be retired on June 30, 2015.</aside> <script> var service_url = 'https://www.googleapis.com/freebase/v1/search'; var params = { 'query': 'Cee Lo Green', 'filter': '(all type:/music/artist created:"The Lady Killer")', 'limit': 10, 'indent': true }; $.getJSON(service_url + '?callback=?', params, function(response) { $.each(response.result, function(i, result) { $('<div>', {text:result['name']}).appendTo(document.body); }); }); </script> </body> </html>This example uses the jQuery library.
PHP
<!DOCTYPE html> <html> <body> <?php include('.freebase-api-key'); $service_url = 'https://www.googleapis.com/freebase/v1/search'; $params = array( 'query' => 'Blue Bottle', 'key' => $freebase_api_key ); $url = $service_url . '?' . http_build_query($params); $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $response = json_decode(curl_exec($ch), true); curl_close($ch); foreach($response['result'] as $result) { echo $result['name'] . '<br/>'; } ?> </body> </html>
Also see the Client Libraries page to see if your favorite language is supported.
Search API documentation
Also see the following documentation:
- See the Search reference documents for details on how to use the API.
- See the Search Cookbook for more information on how to construct detailed search queries.
- See the Search Metaschema document for information on how the relationships between entities and properties are described.
Security considerations
The Search API indexes and searches user generated content stored in the Freebase graph. This means that you cannot directly use the content on a web page without safely escaping it first.
See Getting Started: Security for more information.
Advanced filtering
The Search API supports a large number of filter constraints to better aim the search at the correct entities.
For example, using a "type" filter constraint, we can show a list of the most notable people in Freebase.
filter=(any type:/people/person)
Filter constraints accept a variety of inputs:
- Human readable IDs for schema entities or users, for example:
/people/person
for a type constraint/film
for a domain constraint
- Freebase MIDs, for example:
/m/04kr
for the same/people/person
type constraint/m/010s
for the above/film
domain constraint
- Entity names, for example:
"person"
for a less precise/people/person
type constraint"film"
for a less precise/film
domain constraint
Filter constraints can be classified into a few categories. See the Search Cookbook for more details.
Filter constraints can be freely combined and repeated in the SearchRequest
directly. Repeated filter constraint parameters are combined into an OR query. Different filter constraint parameters or groups are combined into an AND query.
For example:
To search for "people or cities named Gore", try:
query=gore &filter=(any type:/people/person type:/location/citytown)
This combining behavior can be overriden and better controlled with the filter parameter which offers a richer interface to combining constraints. It is an s-expression, possibly arbitrarily nested, where the operator is one of:
any
, logically an ORall
, logically an ANDnot
should
, which can only be used at the top level and which denotes that the constraint is optional. During scoring, matches that don't match optional constraints have their score divided in half for each optional constraint they don't match.
For example:
To match on the /people/person
type or the /film
domain, try:
query=gore &filter=(any type:/people/person domain:/film)